IO — HILSTER’s proprietary IO-hardware
Introduction
HILSTER offers prorietary IO-hardware that is DIN rail mountable. Currently there are four different types and sizes available.
You need the io
feature in your license to unlock the IO hardware features.
For every device an abstraction is available. To read inputs or write outputs
you simply have to assign a value to an output or read an input.
The abstractors offer members with the name of the inputs and outputs, e.g.
io.R6
for relay 6, io.DO0
for digital output 0 or io.AI3
for
analog input 3.
For example to switch on a relay io.R6
you have to assign True
to it.
io.R6 = True
To reset the reley you have to assign False
.
io.R6 = False
To set the digital output pin io.D3
you have to assign True
to it.
io.D3 = True
To reset the digital output pin assign False
to it.
io.R6 = False
To set an analog (pwm) level to 50 % you have to assign an integer value knowing the underlying pwm type. In the following example an 8-bit-pwm is assumed.
io.AO0 = 0xf7 # 50 % pwm level
Finally to read an analog level you have to read you simply have to read an analog input
analog_value = io.AI3
IO Mini
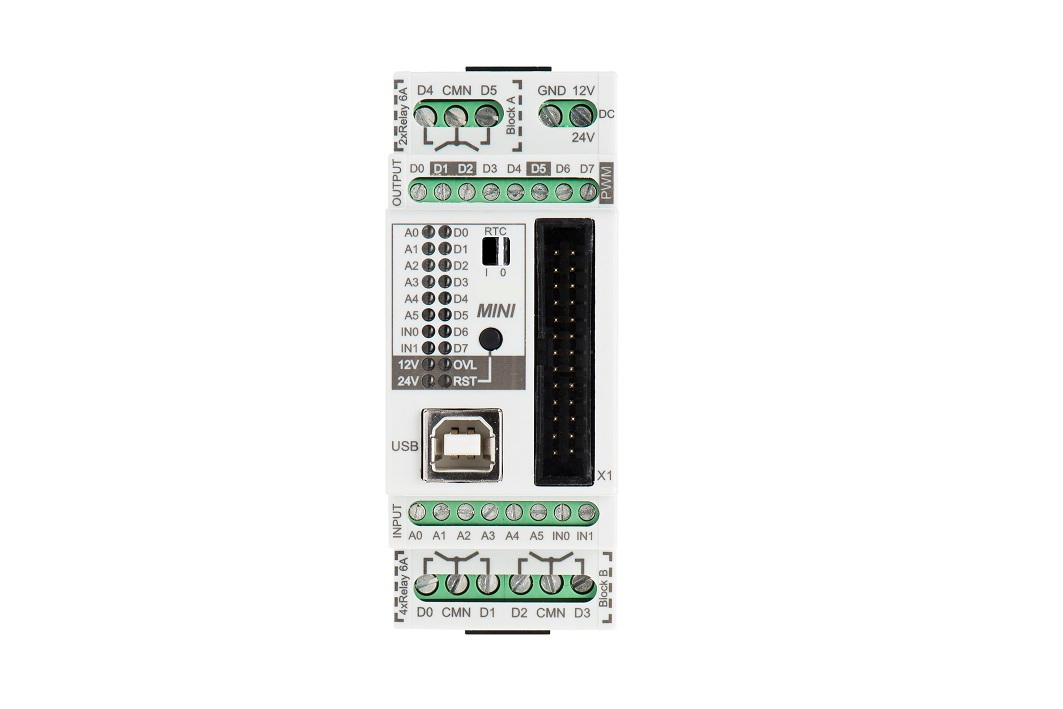
Features:
6 relays (230 V/6 A)
8 analog/digital inputs
4 digital outputs (2 A)
1 UART
1 SPI
1 I2C
RTC
A sample implementation and usage can be found here:
from htf.io import IOMini as IO
io = IO("/dev/ttyACM0")
# set D1
io.D1 = True
# reset D1
io.D1 = False
#read A0
analog_value = io.A0
#read D0
digital_value = io.D0
# etc.
- class htf.io.IOMini(port: str, timeout: int | float = 1.0)
Initialize an IOMini.
- Parameters:
port – the name of the serial connection
timeout=1.0 – the time-out in seconds
- A0
Analog input A0
- A1
Analog input A1
- A2
Analog input A2
- A3
Analog input A3
- A4
Analog input A4
- A5
Analog input A5
- D0
Digital output D0
- D1
Digital output D1
- D2
Digital output D2
- D3
Digital output D3
- D4
Digital output D4
- D5
Digital output D5
- D6
Digital output D6
- D7
Digital output D7
- IN0
Digital input IN0
- IN1
Digital input IN1
IO Maxi
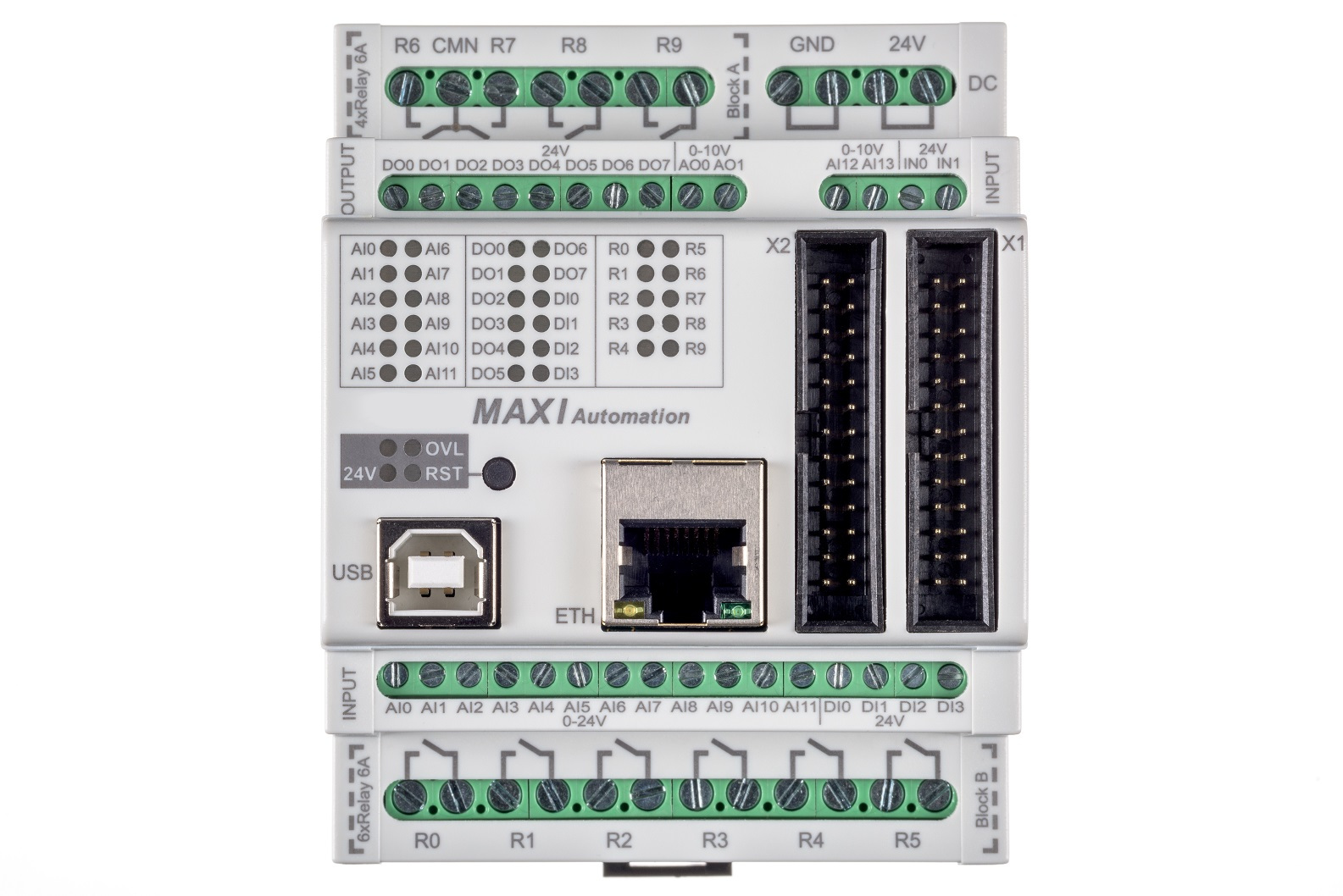
Features:
10 relays (230V/6A)
2 analog outputs (0 to 10 V)
12 analog inputs (24 V)
12 digital inputs (24 V)
8 digital outputs (24 V)
2 UART
1 SPI
1 I2C
RTC
Ethernet
A sample implementation and usage can be found here:
from htf.io import IOMaxi as IO
io = IO("/dev/ttyACM0")
# set R6
io.R6 = True
# reset R6
io.R6 = False
#read AI0
analog_value = io.AI0
#read DI0
digital_value = io.DI0
# etc.
- class htf.io.IOMaxi(port: str, timeout: int | float = 1.0)
Initialize an IOMaxi.
- Parameters:
port – the name of the serial connection
timeout=1.0 – the time-out in seconds
- A0
Analog input A0
- A1
Analog input A1
- A2
Analog input A2
- A3
Analog input A3
- A4
Analog input A4
- A5
Analog input A5
- A6
Analog input A6
- A7
Analog input A7
- A8
Analog input A8
- A9
Analog input A9
- D0
Digital output D0
- D1
Digital output D1
- D10
Digital output D10
- D11
Digital output D11
- D2
Digital output D2
- D3
Digital output D3
- D4
Digital output D4
- D5
Digital output D5
- D6
Digital output D6
- D7
Digital output D7
- D8
Digital output D8
- D9
Digital output D9
- IN0
Digital input IN0
- IN1
Digital input IN1
- R0
Relay R0
- R1
Relay R1
- R2
Relay R2
- R3
Relay R3
- R4
Relay R4
- R5
Relay R5
- R6
Relay R6
- R7
Relay R7
- R8
Relay R8
- R9
Relay R9
IO Maxi Automation
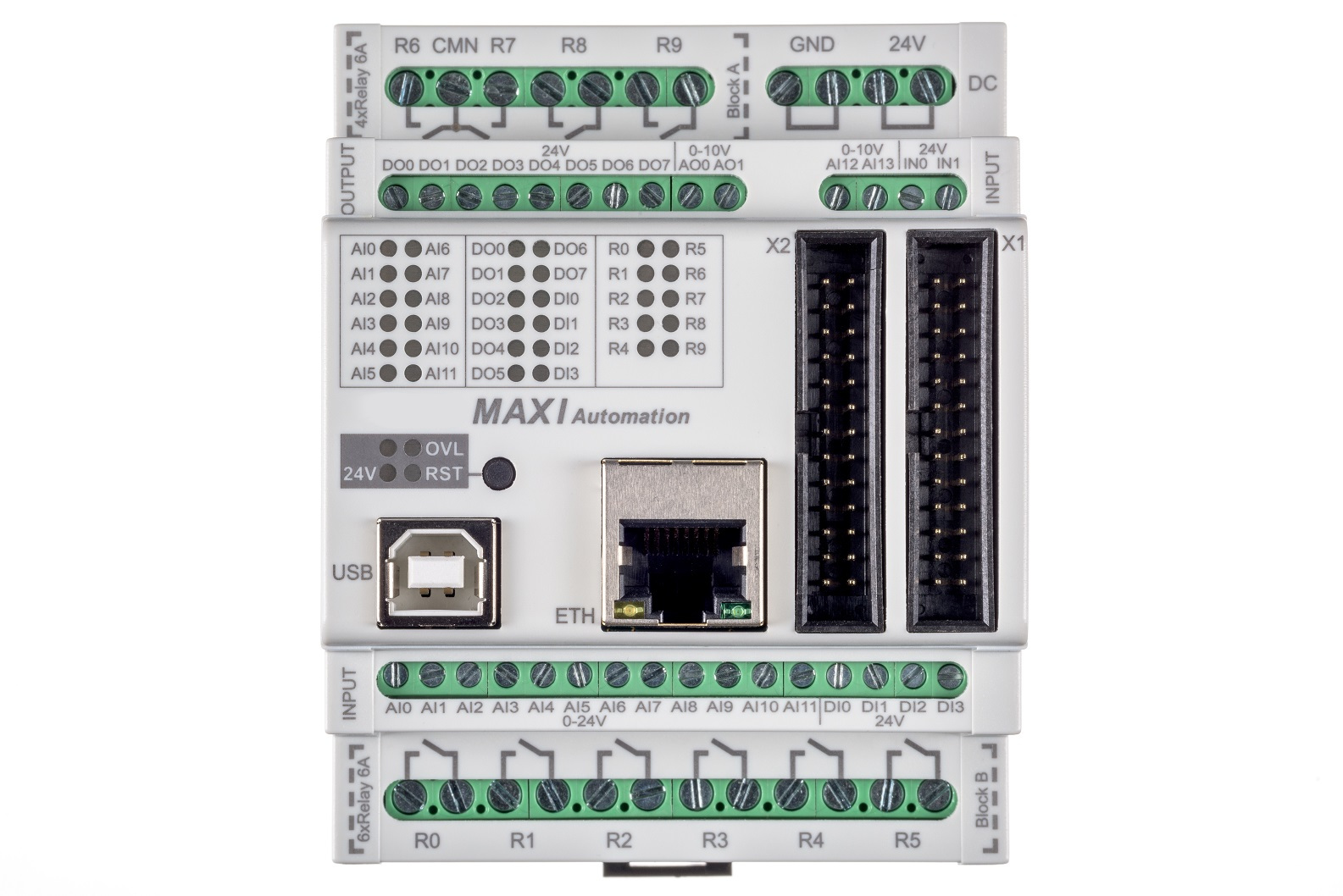
Features:
10 relays (230 V/6 A)
2 analog outputs (0 to 24 V)
2 analog inputs (10 V)
12 analog/digital inputs (24 V)
4 digital inputs (24 V)
8 digital outputs (24 V)
2 UART
1 SPI
1 I2C
RTC
Ethernet
A sample impelentation and usage can be found here:
from htf.io import IOMaxiAutomation as IO
io = IO("/dev/ttyACM0")
# set R6
io.R6 = True
# reset R6
io.R6 = False
#read AI0
analog_value = io.AI0
#read DI0
digital_value = io.DI0
# etc.
- class htf.io.IOMaxiAutomation(port: str, timeout: int | float = 1.0)
Initialize an IOMaxiAutomation.
- Parameters:
port – the name of the serial connection
timeout=1.0 – the time-out in seconds
- AI0
Analog input AI0
- AI1
Analog input AI1
- AI10
Analog input AI10
- AI11
Analog input AI11
- AI12
Analog input AI12
- AI13
Analog input AI13
- AI2
Analog input AI2
- AI3
Analog input AI3
- AI4
Analog input AI4
- AI5
Analog input AI5
- AI6
Analog input AI6
- AI7
Analog input AI7
- AI8
Analog input AI8
- AI9
Analog input AI9
- AO0
Analog output AO0
- AO1
Analog output AO1
- DI0
Digital input DI0
- DI1
Digital input DI1
- DI2
Digital input DI2
- DI3
Digital input DI3
- DO0
Digital output DO0
- DO1
Digital output DO1
- DO2
Digital output DO2
- DO3
Digital output DO3
- DO4
Digital output DO4
- DO5
Digital output DO5
- DO6
Digital output DO6
- DO7
Digital output DO7
- IN1
Digital input IN1
- IN2
Digital input IN2
- R0
Relay R0
- R1
Relay R1
- R2
Relay R2
- R3
Relay R3
- R4
Relay R4
- R5
Relay R5
- R6
Relay R6
- R7
Relay R7
- R8
Relay R8
- R9
Relay R9
IO Mega
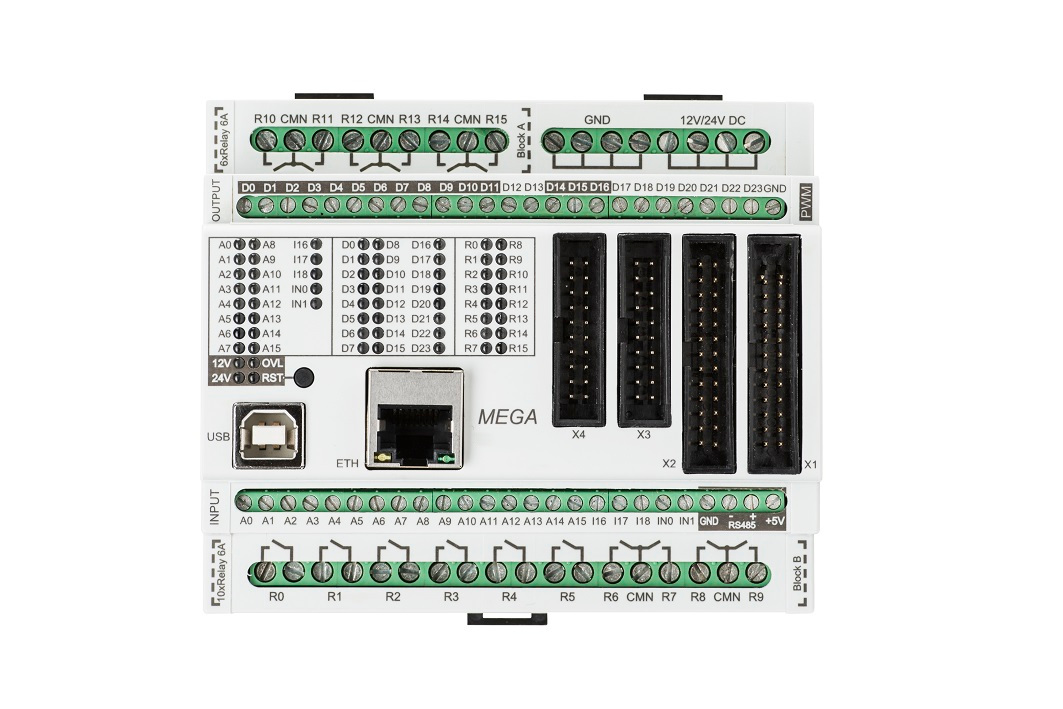
Features:
16 relays (230 V/6 A)
2 analog outputs (0 to 10 V)
21 analog inputs (24 V)
12 digital outputs (24 V)
2 UART
1 RS485
1 SPI
1 I2C
RTC
Ethernet
A sample implementation and usage can be found here:
from htf.io import IOMega as IO
io = IO("/dev/ttyACM0")
# set R6
io.R6 = True
# reset R6
io.R6 = False
#read AI0
analog_value = io.AI0
#read DI0
digital_value = io.DI0
# etc.
- class htf.io.IOMega(port: str, timeout: int | float = 1.0)
Initialize an IOMega.
- Parameters:
port – the name of the serial connection
timeout=1.0 – the time-out in seconds
- A0
Analog input A0
- A1
Analog input A1
- A10
Analog input A10
- A11
Analog input A11
- A12
Analog input A12
- A13
Analog input A13
- A14
Analog input A14
- A15
Analog input A15
- A2
Analog input A2
- A3
Analog input A3
- A4
Analog input A4
- A5
Analog input A5
- A6
Analog input A6
- A7
Analog input A7
- A8
Analog input A8
- A9
Analog input A9
- D0
Digital output D0
- D1
Digital output D1
- D10
Digital output D10
- D11
Digital output D11
- D12
Digital output D12
- D13
Digital output D13
- D14
Digital output D14
- D15
Digital output D15
- D16
Digital output D16
- D17
Digital output D17
- D18
Digital output D18
- D19
Digital output D19
- D2
Digital output D2
- D3
Digital output D3
- D4
Digital output D4
- D5
Digital output D5
- D6
Digital output D6
- D7
Digital output D7
- D8
Digital output D8
- D9
Digital output D9
- I16
Digital input I16
- I17
Digital input I17
- I18
Digital input I18
- IN0
Digital input IN0
- IN1
Digital input IN1
- R0
Relay R0
- R1
Relay R1
- R10
Relay R10
- R11
Relay R11
- R12
Relay R12
- R13
Relay R13
- R14
Relay R14
- R15
Relay R15
- R2
Relay R2
- R3
Relay R3
- R4
Relay R4
- R5
Relay R5
- R6
Relay R6
- R7
Relay R7
- R8
Relay R8
- R9
Relay R9
Changelog
htf-io-4.2.0
add support for Xentara
htf-io-4.1.5
add support for Python 3.13
htf-io-4.1.4
add requirements for validation purposes
htf-io-4.1.3
add build environment data for validation purposes
htf-io-4.1.2
htf-io
can now be used standalone
htf-io-4.1.1
add support for Python 3.12
htf-io-4.1.0
add support for LabJack DAQ devices
htf-io-4.0.0
remove all camel-case methods
add type-hints
use
hlm-3.1
use
oser-3.1
htf-io-3.0.2
add support for Python 3.11
htf-io-3.0.1
fix an import error
htf-io-3.0.0
extract
htf-io
fromhtf