Primitive Types
Primitive types include byte-aligned types like integer numbers, floating point numbers and bit-aligned types like flags, bitfields and also flags.
ByteType
ByteType is an abstract super class which can be used to create byte types.
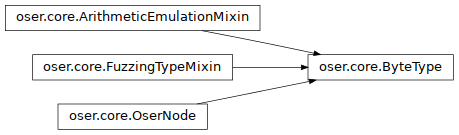
- class oser.ByteType(value: Any = 0, format: str | None = None)
A
ByteType
is an abstract class for byte types.- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Naming Conventions
A type that starts with an "S"
is signed.
A type that starts with an "U"
is unsigned.
A type that has a "L"
as second character is little endian.
A type that has a "B"
as second character is big endian.
8-Bit Types
SLInt8
Signed, little endian 8-Bit integer.
- class oser.SLInt8(value: int = 0, format: str | None = None)
Signed, little endian 8-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SLInt8, to_hex
>>> instance = SLInt8(-10)
>>> print(instance)
-10 (SLInt8)
>>> print(instance.introspect())
0 \xf6 -10 (SLInt8)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\xF6
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
-10 (SLInt8)
SBInt8
Signed, big endian 8-Bit integer.
- class oser.SBInt8(value: int = 0, format: str | None = None)
Signed, big endian 8-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SBInt8, to_hex
>>> instance = SBInt8(-10)
>>> print(instance)
-10 (SBInt8)
>>> print(instance.introspect())
0 \xf6 -10 (SBInt8)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\xF6
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
-10 (SBInt8)
ULInt8
Unsigned, little endian 8-Bit integer.
- class oser.ULInt8(value: int = 0, format: str | None = None)
Unsigned, little endian 8-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import ULInt8, to_hex
>>> instance = ULInt8(10)
>>> print(instance)
10 (ULInt8)
>>> print(instance.introspect())
0 \x0a 10 (ULInt8)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x0A
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
10 (ULInt8)
UBInt8
Unsigned, big endian 8-Bit integer.
- class oser.UBInt8(value: int = 0, format: str | None = None)
Unsigned, big endian 8-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import UBInt8, to_hex
>>> instance = UBInt8(10)
>>> print(instance)
10 (UBInt8)
>>> print(instance.introspect())
0 \x0a 10 (UBInt8)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x0A
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
10 (UBInt8)
Padding
Unsigned 8-Bit integer as a fixed pattern. The pattern to use as a placeholder can be set.
If strict is used the input data is checked while encoding to match the pattern. If input data and pattern do not match a ValueError is raised and decoding is stopped immediately.
- class oser.Padding(value: bytes | str | int = b'\x00', strict: bool = False, format: str | None = None)
Unsigned 8-Bit integer to fill data with a pattern.
- Parameters:
value=b"" – the value to be used as the pattern.
strict – if set to True input data is compared to value. In case of a mismatch a ValueError is raised.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import Padding, to_hex
>>> instance = Padding(value=b"\x00", strict=True)
>>> print(instance)
\x00 (Padding)
>>> print(instance.introspect())
0 \x00 \x00 (Padding)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x00
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
\x00 (Padding)
>>> instance.decode(b"\xff")
oser.DecodeException: Data could not be decoded!
Parsing object is:
0 \xff \xff (Padding)
^^^^ value not allowed: expected \x00 but found \xff
16-Bit Types
SLInt16
Signed, little endian 16-Bit integer.
- class oser.SLInt16(value: int = 0, format: str | None = None)
Signed, little endian 16-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SLInt16, to_hex
>>> instance = SLInt16(-1000)
>>> print(instance)
-1000 (SLInt16)
>>> print(instance.introspect())
0 \x18 -1000 (SLInt16)
1 \xfc
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1
\x18\xFC
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
2
>>> print(instance)
-1000 (SLInt16)
SBInt16
Signed, bit endian 16-Bit integer.
- class oser.SBInt16(value: int = 0, format: str | None = None)
Signed, bit endian 16-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SBInt16, to_hex
>>> instance = SBInt16(-1000)
>>> print(instance)
-1000 (SBInt16)
>>> print(instance.introspect())
0 \xfc -1000 (SBInt16)
1 \x18
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1
\xFC\x18
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
2
>>> print(instance)
-1000 (SBInt16)
ULInt16
Unsigned, little endian 16-Bit integer.
- class oser.ULInt16(value: int = 0, format: str | None = None)
Unsigned, little endian 16-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import ULInt16, to_hex
>>> instance = ULInt16(1000)
>>> print(instance)
1000 (ULInt16)
>>> print(instance.introspect())
0 \xe8 1000 (ULInt16)
1 \x03
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1
\xE8\x03
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
2
>>> print(instance)
1000 (ULInt16)
UBInt16
Unsigned, big endian 16-Bit integer.
- class oser.UBInt16(value: int = 0, format: str | None = None)
Unsigned, big endian 16-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import UBInt16, to_hex
>>> instance = UBInt16(1000)
>>> print(instance)
1000 (UBInt16)
>>> print(instance.introspect())
0 \x03 1000 (UBInt16)
1 \xe8
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1
\x03\xE8
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
2
>>> print(instance)
1000 (UBInt16)
32-Bit Types
SLInt32
Signed, little endian 32-Bit integer.
- class oser.SLInt32(value: int = 0, format: str | None = None)
Signed, little endian 32-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SLInt32, to_hex
>>> instance = SLInt32(-70000)
>>> print(instance)
-70000 (SLInt32)
>>> print(instance.introspect())
0 \x90 -70000 (SLInt32)
1 \xee
2 \xfe
3 \xff
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\x90\xEE\xFE\xFF
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
-70000 (SLInt32)
SBInt32
Signed, big endian 32-Bit integer.
- class oser.SBInt32(value: int = 0, format: str | None = None)
Signed, big endian 32-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SBInt32, to_hex
>>> instance = SBInt32(-70000)
>>> print(instance)
-70000 (SBInt32)
>>> print(instance.introspect())
0 \xff -70000 (SBInt32)
1 \xfe
2 \xee
3 \x90
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\xFF\xFE\xEE\x90
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
-70000 (SBInt32)
ULInt32
Unsigned, little endian 32-Bit integer.
- class oser.ULInt32(value: int = 0, format: str | None = None)
Unsigned, little endian 32-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import ULInt32, to_hex
>>> instance = ULInt32(70000)
>>> print(instance)
70000 (ULInt32)
>>> print(instance.introspect())
0 \x70 70000 (ULInt32)
1 \x11
2 \x01
3 \x00
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\x70\x11\x01\x00
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
70000 (ULInt32)
UBInt32
Unsigned, big endian 32-Bit integer.
- class oser.UBInt32(value: int = 0, format: str | None = None)
Unsigned, big endian 32-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import UBInt32, to_hex
>>> instance = UBInt32(70000)
>>> print(instance)
70000 (UBInt32)
>>> print(instance.introspect())
0 \x00 70000 (UBInt32)
1 \x01
2 \x11
3 \x70
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\x00\x01\x11\x70
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
70000 (UBInt32)
64-Bit Types
SLInt64
Signed, little endian 64-Bit integer.
- class oser.SLInt64(value: int = 0, format: str | None = None)
Signed, little endian 64-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SLInt64, to_hex
>>> instance = SLInt64(-7000000)
>>> print(instance)
-7000000 (SLInt64)
>>> print(instance.introspect())
0 \x40 -7000000 (SLInt64)
1 \x30
2 \x95
3 \xff
4 \xff
5 \xff
6 \xff
7 \xff
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\x40\x30\x95\xFF\xFF\xFF\xFF\xFF
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
-7000000 (SLInt64)
SBInt64
Signed, big endian 64-Bit integer.
- class oser.SBInt64(value: int = 0, format: str | None = None)
Signed, big endian 64-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SBInt64, to_hex
>>> instance = SBInt64(-7000000)
>>> print(instance)
-7000000 (SBInt64)
>>> print(instance.introspect())
0 \xff -7000000 (SBInt64)
1 \xff
2 \xff
3 \xff
4 \xff
5 \x95
6 \x30
7 \x40
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\xFF\xFF\xFF\xFF\xFF\x95\x30\x40
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
-7000000 (SBInt64)
ULInt64
Unsigned, little endian 64-Bit integer.
- class oser.ULInt64(value: int = 0, format: str | None = None)
Unsigned, little endian 64-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import ULInt64, to_hex
>>> instance = ULInt64(7000000)
>>> print(instance)
7000000 (ULInt64)
>>> print(instance.introspect())
0 \xc0 7000000 (ULInt64)
1 \xcf
2 \x6a
3 \x00
4 \x00
5 \x00
6 \x00
7 \x00
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\xC0\xCF\x6A\x00\x00\x00\x00\x00
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
7000000 (ULInt64)
UBInt64
Unsigned, big endian 64-Bit integer.
- class oser.UBInt64(value: int = 0, format: str | None = None)
Unsigned, big endian 64-Bit integer.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import UBInt64, to_hex
>>> instance = UBInt64(7000000)
>>> print(instance)
7000000 (UBInt64)
>>> print(instance.introspect())
0 \x00 7000000 (UBInt64)
1 \x00
2 \x00
3 \x00
4 \x00
5 \x6a
6 \xcf
7 \xc0
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\x00\x00\x00\x00\x00\x6A\xCF\xC0
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
7000000 (UBInt64)
Variable Length Integers
Variable length integers transport 7
bits of information per transferred byte.
The msb of the transferred bytes indicate the last byte of a variable length integer.
All msbs are set except the last one.
Signed values are first converted into an unsigned representation using the zigzag-encoding. This way only one additional bit must be used for the sign.
Zigzag-encoding is done by multiplying a number by 2
. If the number is negative
it is xor’ed by -1
(all bits set) so that all leading sign bits are inverted.
Negative numbers become odd. Positive numbers become even.
original value |
zigzag-encoded value |
---|---|
0 |
0 |
-1 |
1 |
1 |
2 |
-2 |
3 |
2 |
4 |
-3 |
5 |
3 |
6 |
… |
… |
Zigzag-decoding is done by dividing the encoded number by 2
.
If the number was odd it is xor’ed by -1
(all bits set) to convert it back into the normal representation.
UBVarInt
Unsigned, big endian variable length integer.
- class oser.UBVarInt(value: int = 0, format: str | None = None)
Unsigned, big endian variable length integer.
- Parameters:
value=0 – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import UBVarInt, to_hex
>>> instance = UBVarInt(1)
>>> print(instance)
1 (UBVarInt)
>>> print(instance.introspect())
0 \x01 1 (UBVarInt)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x01
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
1 (UBVarInt)
>>> instance.set(150)
>>> print(instance.introspect())
0 \x81 150 (UBVarInt)
1 \x16
>>> instance.set(15000000000)
>>> print(instance.introspect())
0 \x80 15000000000 (UBVarInt)
1 \xb7
2 \xf0
3 \xc7
4 \xac
5 \x00
ULVarInt
Unsigned, little endian variable length integer.
- class oser.ULVarInt(value: int = 0, format: str | None = None)
Unsigned, little endian variable length integer.
- Parameters:
value=0 – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import ULVarInt, to_hex
>>> instance = ULVarInt(1)
>>> print(instance)
1 (ULVarInt)
>>> print(instance.introspect())
0 \x01 1 (ULVarInt)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x01
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
1 (ULVarInt)
>>> instance.set(150)
>>> print(instance.introspect())
0 \x96 150 (ULVarInt)
1 \x01
>>> instance.set(15000000000)
>>> print(instance.introspect())
0 \x80 15000000000 (ULVarInt)
1 \xac
2 \xc7
3 \xf0
4 \xb7
5 \x00
SBVarInt
Signed, big endian variable length integer.
- class oser.SBVarInt(value: int = 0, format: str | None = None)
Signed, big endian variable length integer.
- Parameters:
value=0 – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SBVarInt, to_hex
>>> instance = SBVarInt(-1)
>>> print(instance)
-1 (SBVarInt)
>>> print(instance.introspect())
0 \x01 -1 (SBVarInt)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x01
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
-1 (SBVarInt)
>>> instance.set(150)
>>> print(instance.introspect())
0 \x82 150 (SBVarInt)
1 \x2c
>>> instance.set(-150)
>>> print(instance.introspect())
0 \x82 -150 (SBVarInt)
1 \x2b
>>> instance.set(15000000000)
>>> print(instance.introspect())
0 \x80 15000000000 (SBVarInt)
1 \xef
2 \xe1
3 \x8e
4 \xd8
5 \x00
>>> instance.set(-15000000000)
>>> print(instance.introspect())
0 \x80 -15000000000 (SBVarInt)
1 \xef
2 \xe1
3 \x8e
4 \xd7
5 \x7f
SLVarInt
Signed, little endian variable length integer.
- class oser.SLVarInt(value: int = 0, format: str | None = None)
Signed, little endian variable length integer.
- Parameters:
value=0 – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import SLVarInt, to_hex
>>> instance = SLVarInt(-1)
>>> print(instance)
-1 (SLVarInt)
>>> print(instance.introspect())
0 \x01 -1 (SLVarInt)
>>> binary = instance.encode()
>>> print(to_hex(binary))
0
\x01
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
1
>>> print(instance)
-1 (SLVarInt)
>>> instance.set(150)
>>> print(instance.introspect())
0 \xac 150 (SLVarInt)
1 \x02
>>> instance.set(-150)
>>> print(instance.introspect())
0 \xab -150 (SLVarInt)
1 \x02
>>> instance.set(15000000000)
>>> print(instance.introspect())
0 \x80 15000000000 (SLVarInt)
1 \xd8
2 \x8e
3 \xe1
4 \xef
5 \x00
>>> instance.set(-15000000000)
>>> print(instance.introspect())
0 \xff -15000000000 (SLVarInt)
1 \xd7
2 \x8e
3 \xe1
4 \xef
5 \x00
Float Types
LFloat
Little endian 32-Bit float.
- class oser.LFloat(value: float = 0.0)
Little endian 32-Bit float.
- Parameters:
value – float = 0.0: the initial value.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import LFloat, to_hex
>>> instance = LFloat(-12345)
>>> print(instance)
-12345 (LFloat)
>>> print(instance.introspect())
0 \x00 -12345 (LFloat)
1 \xe4
2 \x40
3 \xc6
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\x00\xE4\x40\xC6
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
-12345.0 (LFloat)
BFloat
Big endian 32-Bit float.
- class oser.BFloat(value: float = 0.0)
Big endian 32-Bit float.
- Parameters:
value – float = 0.0: the initial value.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import BFloat, to_hex
>>> instance = BFloat(-12345)
>>> print(instance)
-12345 (BFloat)
>>> print(instance.introspect())
0 \xc6 -12345 (BFloat)
1 \x40
2 \xe4
3 \x00
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3
\xC6\x40\xE4\x00
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
4
>>> print(instance)
-12345.0 (BFloat)
Double Types
LDouble
Little endian 64-Bit float.
- class oser.LDouble(value: float = 0.0)
Little endian 64-Bit float.
- Parameters:
value – float = 0.0: the initial value.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import LDouble, to_hex
>>> instance = LDouble(-12345)
>>> print(instance)
-12345 (LDouble)
>>> print(instance.introspect())
0 \x00 -12345 (LDouble)
1 \x00
2 \x00
3 \x00
4 \x80
5 \x1c
6 \xc8
7 \xc0
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\x00\x00\x00\x00\x80\x1C\xC8\xC0
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
-12345.0 (LDouble)
BDouble
Big endian 64-Bit float.
- class oser.BDouble(value: float = 0.0)
Big endian 64-Bit float.
- Parameters:
value – float = 0.0: the initial value.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import BDouble, to_hex
>>> instance = BDouble(-12345)
>>> print(instance)
-12345 (BDouble)
>>> print(instance.introspect())
0 \xc0 -12345 (BDouble)
1 \xc8
2 \x1c
3 \x80
4 \x00
5 \x00
6 \x00
7 \x00
>>> binary = instance.encode()
>>> print(to_hex(binary))
0| 1| 2| 3| 4| 5| 6| 7
\xC0\xC8\x1C\x80\x00\x00\x00\x00
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
8
>>> print(instance)
-12345.0 (BDouble)
BitType
oser.BitType
is an abstract super class which can be used to create
bit types.
oser.BitType
should not be used alone but within a oser.BitStruct
as a member since
oser.BitType
decodes and encodes bit-strings and not byte-strings.
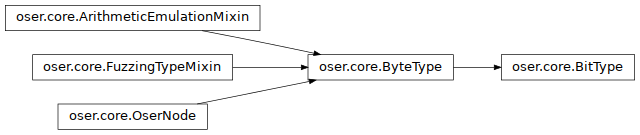
- class oser.BitType(value: Any = 0, format: str | None = None)
A
BitType
is an abstract class for bit types.- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bits that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Bit Types
Flag
1-Bit value used as a flag in a bit context.
- class oser.Flag(value: bool | int = False, format: str | None = None)
1-Bit value used as a flag in a bit context.
- Parameters:
value=False – can be
True
orFalse
.format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bits that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bits that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import Flag
>>> instance = Flag(1)
>>> print(instance)
1 (Flag)
>>> print(instance.introspect())
0.7 1 1 (Flag)
>>> bit_string = instance.encode()
>>> print(bit_string)
1
>>> bitsDecoded = instance.decode(bit_string)
>>> print(bitsDecoded)
1
>>> print(instance)
1 (Flag)
Nibble
4-Bit value in a bit context.
- class oser.Nibble(value: int = 0, format: str | None = None)
4-Bit value in a bit context.
- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bits that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bits that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import Nibble
>>> instance = Nibble(7)
>>> print(instance)
7 (Nibble)
>>> print(instance.introspect())
0.7 0 7 (Nibble)
0.6 1
0.5 1
0.4 1
>>> bit_string = instance.encode()
>>> print(bit_string)
0111
>>> bitsDecoded = instance.decode(bit_string)
>>> print(bitsDecoded)
4
>>> print(instance)
7 (Nibble)
BitField
N-Bit value with a variable length in a bit context.
- class oser.BitField(value: int = 0, length: int = 1, format: str | None = None)
N-Bit value with a variable length in a bit context.
Initialize a
BitField
.- Parameters:
value=0 – the initial value
length=1 – the length in bits
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bits that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bits that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import BitField
>>> instance = BitField(value=0xabc, length=12)
>>> print(instance)
2748 (BitField(12))
>>> print(instance.introspect())
0.7 1 2748 (BitField(12))
0.6 0
0.5 1
0.4 0
0.3 1
0.2 0
0.1 1
0.0 1
1.7 1
1.6 1
1.5 0
1.4 0
>>> bit_string = instance.encode()
>>> print(bit_string)
101010111100
>>> bitsDecoded = instance.decode(bit_string)
>>> print(bitsDecoded)
12
>>> print(instance)
2748 (BitField(12))
PaddingFlag
1-Bit padding value used as a flag in a bit context. Value is fixed and may be strict. If strict is set the input data and the value are compared with each other. If the values differe a ValueError is raised.
- class oser.PaddingFlag(value: bool = False, strict: bool = False, format: str | None = None)
PaddingFlag is a one bit padding.
- Parameters:
value=False – can be
True
orFalse
.strict=False – if set to
True
an exception is raised when decoded data does not matchvalue
.format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bits that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bits that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import PaddingFlag
>>> instance = PaddingFlag(value=1, strict=True)
>>> print(instance)
1 (PaddingFlag)
>>> print(instance.introspect())
0.7 1 1 (PaddingFlag)
>>> bit_string = instance.encode()
>>> print(bit_string)
1
>>> bitsDecoded = instance.decode(bit_string)
>>> print(bitsDecoded)
1
>>> print(instance)
1 (PaddingFlag)
>>> instance.decode(b"0")
Exception: Padding error: expected 1 got 0!
Null
A null object. It does not lead to any data but can be used as a placeholder or in case of an unused else branch, etc.
- class oser.Null
A null-object that does not contain any data.
- Parameters:
value – the initial value.
format=None (string) – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded (always 0).
- Parameters:
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string. This is always an empty string.
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None (object) – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values (iterable) – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import Null, to_hex
>>> instance = Null()
>>> print(instance)
Null (Null)
>>> print(instance.introspect())
- - Null
>>> binary = instance.encode()
>>> print(to_hex(binary))
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
0
>>> print(instance)
Null (Null)
Nothing
An invisible object. It can be used to hide members in special conditions.
- class oser.Nothing
Nothing
does not appear in the encoded data and not in the string or introspection.- Parameters:
value – the initial value.
format=None – Use “hex” to format values to hexadecimal. Use “bin” to format values to binary.
- decode(data: bytes, full_data: bytes = b'', context_data: bytes = b'') int
Decode a binary string and return the number of bytes that were decoded.
- Parameters:
data – the data buffer that is decoded.
full_data – the binary data string until the part to be decoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the number of bytes that were decoded.
- Return type:
- encode(full_data: bytes = b'', context_data: bytes = b'') bytes
Return the encoded binary string. This is always an empty string.
- Parameters:
full_data – the binary data string until the part to be encoded. The user normally does not need to supply this.
context_data – the binary data of the current context. The user normally does not need to supply this.
- Returns:
the encoded binary string.
- Return type:
- get_byte_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- get_size() int
Return the length of the byte type in bytes.
- Returns:
the length of the byte type in bytes.
- Return type:
- introspect(stop_at: ByteStruct | BitStruct | ByteType | BitType | None = None) str
Return the introspection representation of the object as a string.
- Parameters:
stop_at=None – stop introspection at
stop_at
.
- root() ByteStruct | BitStruct
return root element
- set_fuzzing_values(values: Generator[Any, None, None] | List[Any] | None) None
Set fuzzing values.
- Parameters:
values – the values used for fuzzing.
- up() ByteStruct | BitStruct
Return the parent element.
Usage:
>>> from oser import Nothing, to_hex
>>> instance = Nothing()
>>> print(instance)
>>> print(instance.introspect())
>>> binary = instance.encode()
>>> print(to_hex(binary))
>>> bytes_decoded = instance.decode(binary)
>>> print(bytes_decoded)
0
>>> print(instance)